1. Basic usage
DustPy
is using on the simframe
framework for running scientific simulations. For a detailed description of the usage of simframe
please have a look at the Simframe Documentation.
This notebook demonstrates how to run the most simple DustPy
model, i.e., the model that is run by default, how to plot data, and how to resume simulations from dump files.
The Simulation Frame
To set up a model we have to import the Simulation
class from the DustPy
package.
[1]:
from dustpy import Simulation
We can now create an instance of this class.
[2]:
sim = Simulation()
At this stage sim
is an empty simulation object that controls our simulation.
[3]:
sim
[3]:
DustPy
------
dust : Group (Dust quantities)
gas : Group (Gas quantities)
grid : Group (Grid quantities)
star : Group (Stellar quantities)
-----
t : NoneType
-----
Integrator : not specified
Writer : not specified
All the fields are initialized with None
. All attributes can be easiliy addressed via e.g.
[4]:
sim.gas
[4]:
Group (Gas quantities)
----------------------
boundary : Group (Boundary conditions)
S : Group (Source terms)
torque : Group (Torque parameters)
v : Group (Velocities)
-----
alpha : NoneType
cs : NoneType
eta : NoneType
Fi : NoneType
Hp : NoneType
mfp : NoneType
mu : NoneType
n : NoneType
nu : NoneType
P : NoneType
rho : NoneType
Sigma : NoneType
SigmaFloor : NoneType
T : NoneType
-----
Initializing
We can now initialize the sim
object with Simulation.initialize()
. DustPy
will then fill all the fields with default values.
[5]:
sim.initialize()
sim
object has now values assigned to its fields.[6]:
sim.gas
[6]:
Group (Gas quantities)
----------------------
boundary : Group (Boundary conditions)
S : Group (Source terms)
torque : Group (Torque parameters)
v : Group (Velocities)
-----
alpha : Field (Turbulent alpha parameter)
cs : Field (Isothermal sound speed [cm/s])
eta : Field (Pressure gradient parameter)
Fi : Field (Gas flux interfaces [g/cm/s])
Hp : Field (Pressure scale height [cm])
mfp : Field (Midplane mean free path [cm])
mu : Field (Mean molecular weight [g])
n : Field (Miplane number density [1/cm³])
nu : Field (Kinematic viscosity [cm²/s])
P : Field (Midplane pressure [g/cm/s²])
rho : Field (Miplane mass density [g/cm³])
Sigma : Field (Surface density [g/cm²])
SigmaFloor : Field (Floor value of surface density [g/cm²])
T : Field (Temperature [K])
-----
You can also display the full table of contents of the sim
object.
[7]:
sim.toc
DustPy
- dust: Group (Dust quantities)
- a: Field (Particle size [cm])
- backreaction: Group (Backreaction coefficients)
- A: Field (Pull factor)
- B: Field (Push factor)
- boundary: Group (Boundary conditions)
- inner: Constant gradient
- outer: Value
- coagulation: Group (Coagulation quantities)
- A: Field (Fragment normalization factors), constant
- eps: Field (Remnant mass distribution), constant
- lf_ind: Field (Index of largest fragment), constant
- phi: Field (Fragment distribution), constant
- rm_ind: Field (Smaller index of remnant), constant
- stick: Field (Sticking matrix), constant
- stick_ind: Field (Non-zero elements of sticking matrix), constant
- D: Field (Diffusivity [cm²/s])
- delta: Group (Mixing parameters)
- rad: Field (Radial mixing parameter)
- turb: Field (Turbulent mixing parameter)
- vert: Field (Vertical mixing parameter)
- eps: Field (Dust-to-gas ratio)
- Fi: Group (Fluxes)
- adv: Field (Advective flux [g/cm/s])
- diff: Field (Diffusive flux [g/cm/s])
- tot: Field (Total flux [g/cm/s])
- fill: Field (Filling factor)
- H: Field (Scale heights [cm])
- kernel: Field (Collision kernel [cm²/s])
- p: Group (Probabilities)
- frag: Field (Fragmentation probability)
- stick: Field (Sticking probability)
- rho: Field (Midplane mass density per mass bin [g/cm³])
- rhos: Field (Solid state density [g/cm³])
- S: Group (Sources)
- coag: Field (Coagulation sources [g/cm²/s])
- ext: Field (External sources [g/cm²/s])
- hyd: Field (Hydrodynamic sources [g/cm²/s])
- tot: Field (Tot sources [g/cm²/s])
- Sigma: Field (Surface density per mass bin [g/cm²])
- SigmaFloor: Field (Floor value of surface density [g/cm²])
- St: Field (Stokes number)
- v: Group (Velocities)
- driftmax: Field (Maximum drift velocity [cm/s])
- frag: Field (Fragmentation velocity [cm/s])
- rad: Field (Radial velocity [cm/s])
- rel: Group (Relative velocities)
- azi: Field (Relative azimuthal velocity [cm/s])
- brown: Field (Relative Brownian motion velocity [cm/s])
- rad: Field (Relative radial velocity [cm/s])
- tot: Field (Total relative velocity [cm/s])
- turb: Field (Relative turbulent velocity [cm/s])
- vert: Field (Relative vertical settling velocity [cm/s])
- gas: Group (Gas quantities)
- alpha: Field (Turbulent alpha parameter)
- boundary: Group (Boundary conditions)
- inner: Constant gradient
- outer: Value
- cs: Field (Isothermal sound speed [cm/s])
- eta: Field (Pressure gradient parameter)
- Fi: Field (Gas flux interfaces [g/cm/s])
- Hp: Field (Pressure scale height [cm])
- mfp: Field (Midplane mean free path [cm])
- mu: Field (Mean molecular weight [g])
- n: Field (Miplane number density [1/cm³])
- nu: Field (Kinematic viscosity [cm²/s])
- P: Field (Midplane pressure [g/cm/s²])
- rho: Field (Miplane mass density [g/cm³])
- S: Group (Source terms)
- ext: Field (External sources [g/cm²/s])
- hyd: Field (Hydrodynamic sources [g/cm²/s])
- tot: Field (Total sources [g/cm²/s])
- Sigma: Field (Surface density [g/cm²])
- SigmaFloor: Field (Floor value of surface density [g/cm²])
- T: Field (Temperature [K])
- torque: Group (Torque parameters)
- Lambda: Field (Specific angular momentum injection rate by torque [cm²/s²])
- v: Field (Effective velocity imposed by torque [cm/s])
- v: Group (Velocities)
- rad: Field (Radial velocity [cm/s])
- visc: Field (Viscous accretion velocity [cm/s])
- grid: Group (Grid quantities)
- A: Field (Radial grid annulus area [cm²]), constant
- m: Field (Mass grid [g]), constant
- Nm: Field (# of mass bins), constant
- Nr: Field (# of radial grid cells), constant
- OmegaK: Field (Keplerian frequency [1/s])
- r: Field (Radial grid cell centers [cm]), constant
- ri: Field (Radial grid cell interfaces [cm]), constant
- star: Group (Stellar quantities)
- L: Field (Luminosity [erg/s])
- M: Field (Mass [g])
- R: Field (Radius [cm])
- T: Field (Effective temperature [K])
- t: IntVar (Time [s]), Integration variable
Running a Simulation
The simulation is now ready to go. This will take a few minutes. The default simulation is running for 100,000 years.
[8]:
sim.run()
DustPy v1.0.8
Documentation: https://stammler.github.io/dustpy/
PyPI: https://pypi.org/project/dustpy/
GitHub: https://github.com/stammler/dustpy/
Please cite Stammler & Birnstiel (2022).
Checking for mass conservation...
- Sticking:
max. rel. error: 2.81e-14
for particle collision
m[114] = 1.93e+04 g with
m[116] = 3.73e+04 g
- Full fragmentation:
max. rel. error: 6.66e-16
for particle collision
m[90] = 7.20e+00 g with
m[95] = 3.73e+01 g
- Erosion:
max. rel. error: 1.78e-15
for particle collision
m[110] = 5.18e+03 g with
m[118] = 7.20e+04 g
Creating data directory data.
Writing file data/data0000.hdf5
Writing dump file data/frame.dmp
Writing file data/data0001.hdf5
Writing dump file data/frame.dmp
Writing file data/data0002.hdf5
Writing dump file data/frame.dmp
Writing file data/data0003.hdf5
Writing dump file data/frame.dmp
Writing file data/data0004.hdf5
Writing dump file data/frame.dmp
Writing file data/data0005.hdf5
Writing dump file data/frame.dmp
Writing file data/data0006.hdf5
Writing dump file data/frame.dmp
Writing file data/data0007.hdf5
Writing dump file data/frame.dmp
Writing file data/data0008.hdf5
Writing dump file data/frame.dmp
Writing file data/data0009.hdf5
Writing dump file data/frame.dmp
Writing file data/data0010.hdf5
Writing dump file data/frame.dmp
Writing file data/data0011.hdf5
Writing dump file data/frame.dmp
Writing file data/data0012.hdf5
Writing dump file data/frame.dmp
Writing file data/data0013.hdf5
Writing dump file data/frame.dmp
Writing file data/data0014.hdf5
Writing dump file data/frame.dmp
Writing file data/data0015.hdf5
Writing dump file data/frame.dmp
Writing file data/data0016.hdf5
Writing dump file data/frame.dmp
Writing file data/data0017.hdf5
Writing dump file data/frame.dmp
Writing file data/data0018.hdf5
Writing dump file data/frame.dmp
Writing file data/data0019.hdf5
Writing dump file data/frame.dmp
Writing file data/data0020.hdf5
Writing dump file data/frame.dmp
Writing file data/data0021.hdf5
Writing dump file data/frame.dmp
Execution time: 0:08:05
By default DustPy
has written output files into the data/
directory.
Plotting
DustPy
is coming with a simple plotting script that can be used to check the status of a simulation.
[9]:
from dustpy import plot
The plotting script does either take the simulation object as argument or a data directory.
If the argument is a simulation object the script is only plotting the current state.
[10]:
plot.panel(sim)
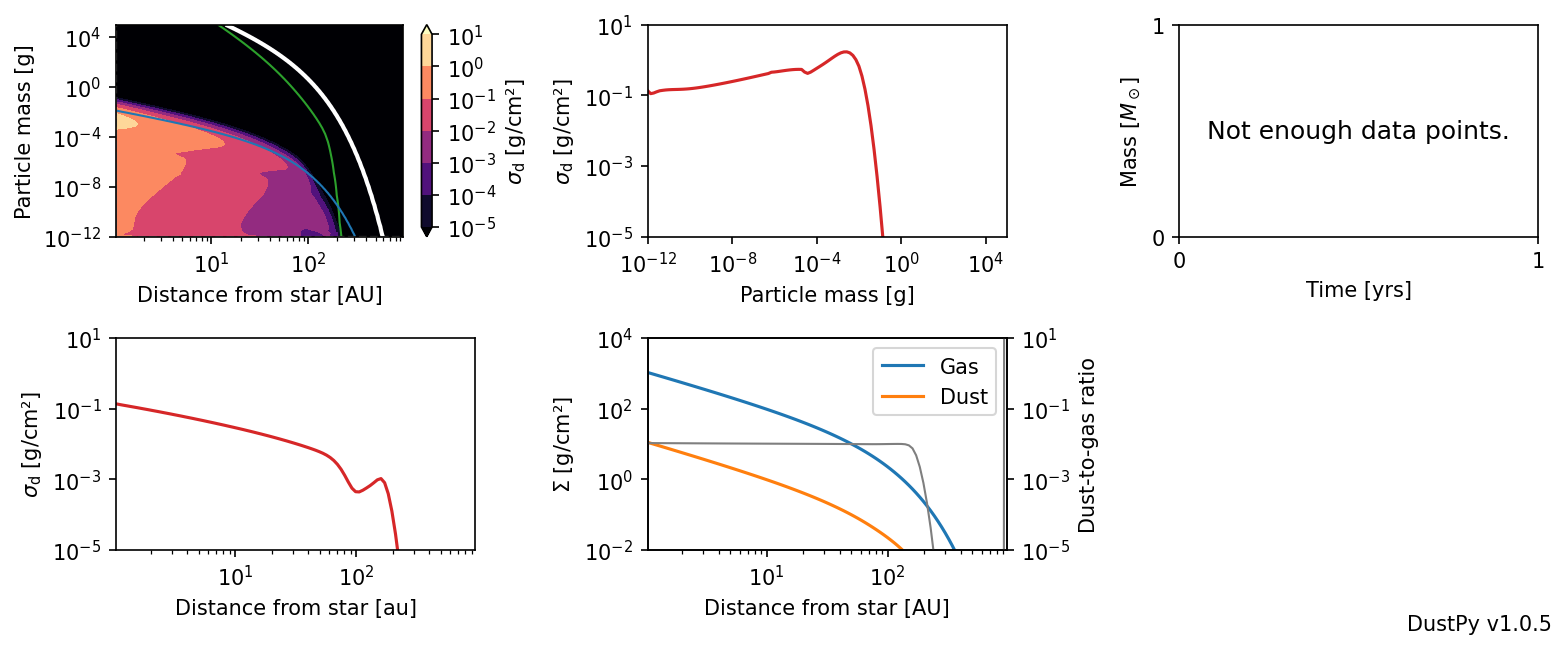
The blue and the green lines in the top left panel are analytical estimates for the fragmentation and drift barrier taken from Birnstiel et al. (2012).
it
, radial ir
, or mass im
index.[11]:
plot.panel("data", it=10, ir=10, im=5)
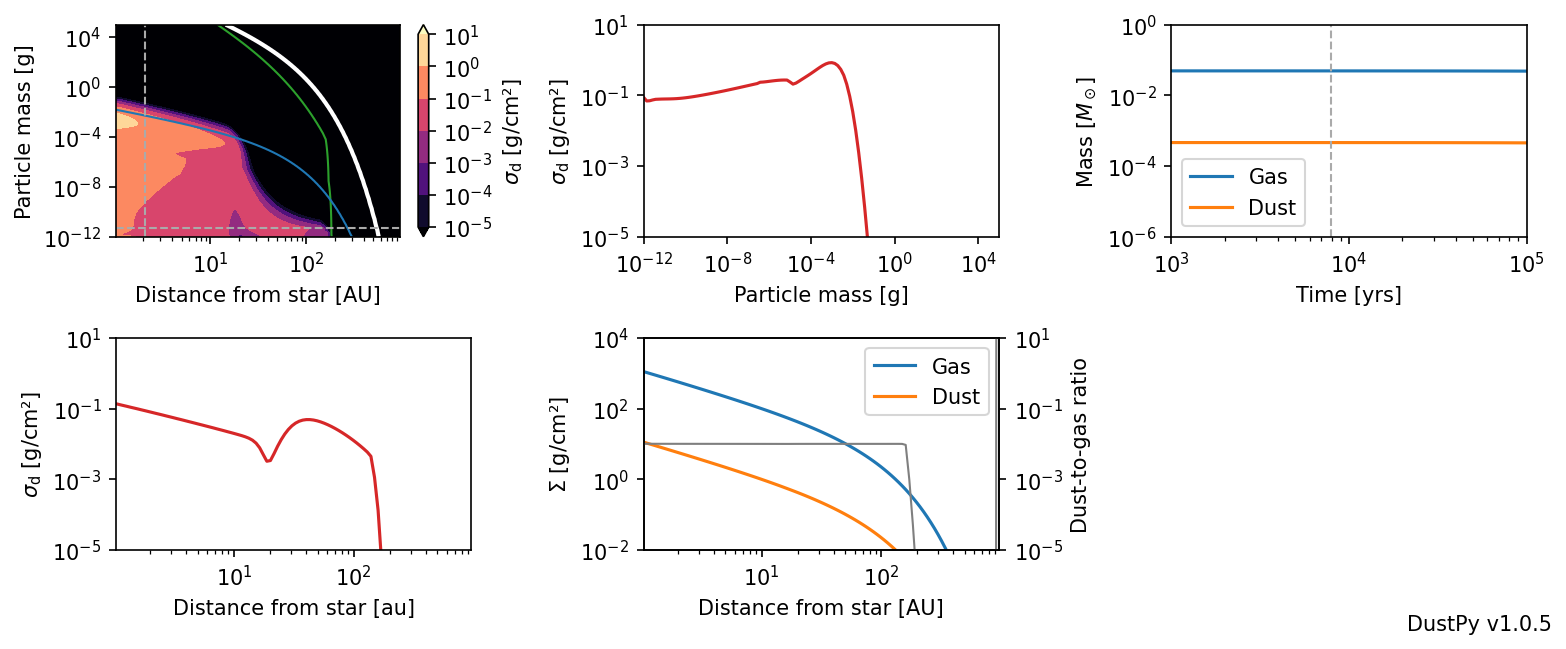
The top middle and bottom left panels show the dust density distribution along the gray dashed lines in the top left panel.
It’s furthermore possible to use an interactive plotting script.
[12]:
plot.ipanel("data")
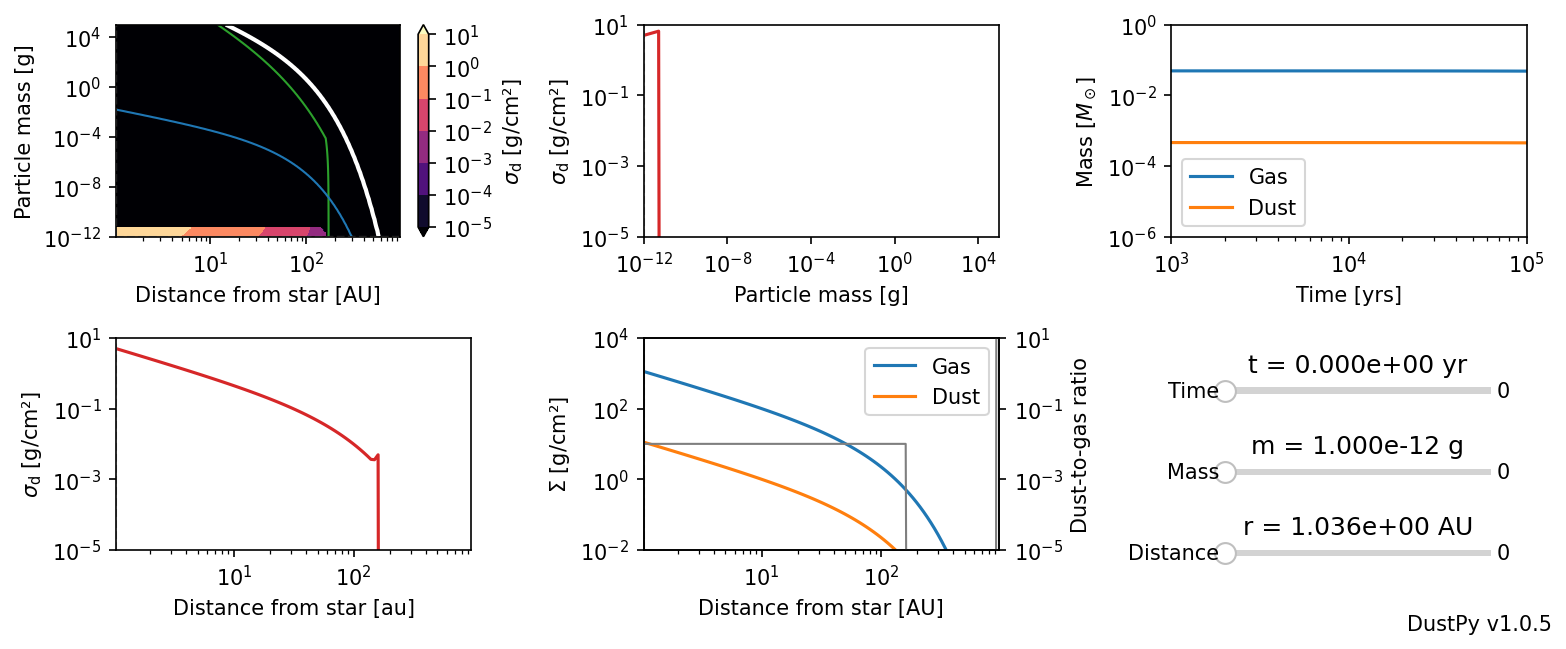
Code Units
The dust density distribution plotted here is given by
In this way the distribution is independent of the mass grid.
The code units DustPy
uses are
meaning the numerical sum over the mass dimension is the dust surface density.
[13]:
sim.dust.Sigma.sum(-1)
[13]:
[1.13955861e+01 1.06029050e+01 9.86083808e+00 9.18342846e+00
8.55022197e+00 7.95880724e+00 7.40751570e+00 6.89262940e+00
6.41213859e+00 5.96470789e+00 5.54702249e+00 5.15740967e+00
4.79485333e+00 4.45647616e+00 4.14091226e+00 3.84740118e+00
3.57350578e+00 3.31813842e+00 3.08071664e+00 2.85916562e+00
2.65262063e+00 2.46064418e+00 2.28150437e+00 2.11451740e+00
1.95934379e+00 1.81456124e+00 1.67962562e+00 1.55425715e+00
1.43731042e+00 1.32834830e+00 1.22712224e+00 1.13273218e+00
1.04482559e+00 9.63172179e-01 8.87107626e-01 8.16575562e-01
7.50810864e-01 6.89621120e-01 6.32894711e-01 5.80091455e-01
5.31029141e-01 4.85553045e-01 4.43312053e-01 4.04219195e-01
3.67924074e-01 3.34411005e-01 3.03318194e-01 2.74567585e-01
2.48051576e-01 2.23579249e-01 2.01057959e-01 1.80325606e-01
1.61301928e-01 1.43852558e-01 1.27896164e-01 1.13322999e-01
1.00046535e-01 8.79948225e-02 7.70548384e-02 6.71860988e-02
5.83447985e-02 5.04684074e-02 4.35164251e-02 3.74032401e-02
3.19732799e-02 2.71302890e-02 2.28306521e-02 1.90507248e-02
1.57598587e-02 1.29208840e-02 1.04939747e-02 8.43870733e-03
6.71307059e-03 5.27059343e-03 4.04552925e-03 2.93217315e-03
1.80167290e-03 6.75066389e-04 1.53949568e-04 2.13652478e-05
1.94975065e-06 1.30896336e-07 6.67074008e-09 2.62519776e-10
8.02115795e-12 1.89028448e-13 3.36492577e-15 4.35408217e-17
3.86257400e-19 2.17471591e-21 7.12064220e-24 1.26262507e-26
1.14601912e-28 9.00875506e-29 7.84588143e-29 6.83347698e-29
5.95170965e-29 5.18372240e-29 4.51483347e-29 3.93225557e-29]
To convert this into
Since the mass grid is strictly logarithmic the following relation holds
The grid constant
[14]:
import numpy as np
A = np.mean(sim.grid.m[1:]/sim.grid.m[:-1])
A
[14]:
1.3894954943731377
We further assume that the mass bin center is exactly in the middle between the mass bin interfaces
Solving for the interfaces yields
We therefore have
and
[15]:
B = 2 * (A-1) / (A+1)
[16]:
sim.dust.Sigma / B
[16]:
[[1.19204136e-01 9.84350200e-02 1.02953636e-01 ... 1.52526930e-23
2.11935481e-23 2.94483396e-23]
[1.13872794e-01 9.37359521e-02 9.78118256e-02 ... 1.32845402e-23
1.84588088e-23 2.56484316e-23]
[1.09085035e-01 8.94843428e-02 9.31369308e-02 ... 1.15703508e-23
1.60769503e-23 2.23388501e-23]
...
[4.45718657e-46 6.19324066e-46 8.60547999e-46 ... 2.30858850e-29
3.20777332e-29 4.45718657e-29]
[3.88204722e-46 5.39408712e-46 7.49505975e-46 ... 2.01069653e-29
2.79385376e-29 3.88204722e-29]
[3.38112178e-46 4.69805348e-46 6.52792414e-46 ... 1.75124347e-29
2.43334490e-29 3.38112178e-29]]
Reading data files
If you want to read data files you can use the read/writer module provided by simframe
, that is used to write the data.
[17]:
from dustpy import hdf5writer
wrtr = hdf5writer()
Make sure that the correct data directory is assigned to the writer.
[18]:
wrtr
[18]:
Writer (HDF5 file format using h5py)
------------------------------------
Data directory : data
File names : data/data0000.hdf5
Overwrite : False
Dumping : True
Options : {'com': 'lzf', 'comopts': None}
Verbosity : 1
You can now read a single data file with
[19]:
data0003 = wrtr.read.output(3)
This function returns a namespace and the data can simply be accessed in the same way as for the Simulation
object.
[20]:
data0003.gas.Sigma
[20]:
array([1.11482110e+003, 1.03983953e+003, 9.69821741e+002, 9.04227215e+002,
8.42832109e+002, 7.85420755e+002, 7.31777194e+002, 6.81682992e+002,
6.34920030e+002, 5.91275189e+002, 5.50544320e+002, 5.12534493e+002,
4.77064725e+002, 4.43965816e+002, 4.13079785e+002, 3.84259188e+002,
3.57366435e+002, 3.32273135e+002, 3.08859484e+002, 2.87013695e+002,
2.66631460e+002, 2.47615457e+002, 2.29874880e+002, 2.13325007e+002,
1.97886797e+002, 1.83486508e+002, 1.70055346e+002, 1.57529137e+002,
1.45848019e+002, 1.34956154e+002, 1.24801461e+002, 1.15335366e+002,
1.06512569e+002, 9.82908230e+001, 9.06307359e+001, 8.34955752e+001,
7.68510926e+001, 7.06653567e+001, 6.49085983e+001, 5.95530643e+001,
5.45728817e+001, 4.99439298e+001, 4.56437205e+001, 4.16512862e+001,
3.79470742e+001, 3.45128472e+001, 3.13315906e+001, 2.83874240e+001,
2.56655183e+001, 2.31520177e+001, 2.08339655e+001, 1.86992343e+001,
1.67364592e+001, 1.49349763e+001, 1.32847624e+001, 1.17763800e+001,
1.04009246e+001, 9.14997520e+000, 8.01554894e+000, 6.99005824e+000,
6.06627221e+000, 5.23728154e+000, 4.49646740e+000, 3.83747456e+000,
3.25418891e+000, 2.74071962e+000, 2.29138599e+000, 1.90070920e+000,
1.56340881e+000, 1.27440389e+000, 1.02881847e+000, 8.21990813e-001,
6.49485768e-001, 5.07109402e-001, 3.90924812e-001, 2.97267968e-001,
2.22762311e-001, 1.64330846e-001, 1.19204531e-001, 8.49259450e-002,
5.93474779e-002, 4.06236257e-002, 2.71973772e-002, 1.77811047e-002,
1.13327688e-002, 7.02859232e-003, 4.23358844e-003, 2.47143530e-003,
1.39513957e-003, 7.59751946e-004, 3.98102772e-004, 2.00166527e-004,
9.62894557e-005, 4.41757146e-005, 1.92634657e-005, 7.95526294e-006,
3.09923559e-006, 1.13428831e-006, 3.87432578e-007, 1.00000000e-100])
You can also read the full data directory with
[21]:
data = wrtr.read.all()
The data has now an additional dimension for time.
[22]:
data.gas.Sigma.shape
[22]:
(22, 100)
Data files can be quite large and reading the entire data set can take some time. Is is also possible to only read a single field from the data files instead of the entire data set.
[23]:
SigmaGas = wrtr.read.sequence("gas.Sigma")
[24]:
SigmaGas.shape
[24]:
(22, 100)
It is also possible to exclude certain fields from being written into the data files to save memory by setting their save
attribute to False
.
[25]:
sim.dust.kernel.save = False
There is a utility function implemented in DustPy
that is loading all data fields needed for the default plots including an estimate for the growth barriers. The function read_data()
either takes the simulation object sim
or a path to the data directory as input and returns a SimpleNamespace
object with the data fields.
[26]:
from dustpy.utils import read_data
[27]:
data = read_data("data")
For example the Stokes numbers of fragmentation limited particles of shape (Nt, Nr)
that can be used to plot growth limits.
[28]:
data.dust.St_limits.frag
[28]:
array([[0.00042705, 0.00044205, 0.00045759, ..., 0.012177 , 0.01260506,
0.01304818],
[0.00042705, 0.00044205, 0.00045759, ..., 0.012177 , 0.01260506,
0.01304818],
[0.00042705, 0.00044205, 0.00045759, ..., 0.012177 , 0.01260506,
0.01304818],
...,
[0.00042705, 0.00044205, 0.00045759, ..., 0.012177 , 0.01260506,
0.01304818],
[0.00042705, 0.00044205, 0.00045759, ..., 0.012177 , 0.01260506,
0.01304818],
[0.00042705, 0.00044205, 0.00045759, ..., 0.012177 , 0.01260506,
0.01304818]], shape=(22, 100))
Reading Dump Files
The data files are only containing the pure data, but no information about the operations DustPy
has to perform, like customized functions. Therefore, it’s not possible to directly restart a simulation from data files.
simframe
is saving by default the most recent dump file, from which a simulation can be restarted.
Attention: Malware can be injected with dump files, which are pickled objects. Only read dump files that you have created yourself or from sources that you trust! Dump files have to be read with the same version of DustPy
as they were written. Otherwise, it is not guaranteed to work.
[29]:
from dustpy import readdump
[30]:
sim_restart = readdump("data/frame.dmp")
This is now a simulation frame that should be identical to our previous object.
[31]:
sim.gas.Sigma == sim_restart.gas.Sigma
[31]:
[ True True True True True True True True True True True True
True True True True True True True True True True True True
True True True True True True True True True True True True
True True True True True True True True True True True True
True True True True True True True True True True True True
True True True True True True True True True True True True
True True True True True True True True True True True True
True True True True True True True True True True True True
True True True True]
We can now, for example, add more snapshots and restart the simulation. Here we just want to extend the run by one year.
[32]:
from dustpy import constants as c
sim_restart.t.snapshots = np.hstack((sim_restart.t.snapshots, [100001.*c.year]))
The current time is
[33]:
sim_restart.t / c.year
[33]:
100000.0
We can now restart the simulation for another year.
[34]:
sim_restart.run()
DustPy v1.0.8
Documentation: https://stammler.github.io/dustpy/
PyPI: https://pypi.org/project/dustpy/
GitHub: https://github.com/stammler/dustpy/
Please cite Stammler & Birnstiel (2022).
Checking for mass conservation...
- Sticking:
max. rel. error: 2.81e-14
for particle collision
m[114] = 1.93e+04 g with
m[116] = 3.73e+04 g
- Full fragmentation:
max. rel. error: 6.66e-16
for particle collision
m[90] = 7.20e+00 g with
m[95] = 3.73e+01 g
- Erosion:
max. rel. error: 1.78e-15
for particle collision
m[110] = 5.18e+03 g with
m[118] = 7.20e+04 g
Writing file data/data0022.hdf5
Writing dump file data/frame.dmp
Execution time: 0:00:01
Another file was written and the current time is now
[35]:
sim_restart.t / c.year
[35]:
100001.0