2. Simple Customization
If you do not want to run the standard simulation you can easily modify the initial conditions.
[1]:
from dustpy import Simulation
[2]:
sim = Simulation()
The simulation object has an attribute to set the initial conditions Simulation.ini
. The attribute is structured in initial conditions for dust, gas, the grid, and the central star. If you call Simulatio.initialize()
, the simulation object will be filled according the parameters set here. We’ll go through all options one by one.
–> All quantities are in cgs units! <–
[3]:
sim.ini
[3]:
namespace(dust=namespace(aIniMax=0.0001,
allowDriftingParticles=False,
erosionMassRatio=10.0,
d2gRatio=0.01,
distExp=-3.5,
excavatedMass=1.0,
fragmentDistribution=-1.8333333333333333,
rhoMonomer=1.67,
vFrag=100.0),
gas=namespace(alpha=0.001,
Mdisk=9.942049353490256e+31,
mu=3.847030424486999e-24,
SigmaExp=-1.0,
SigmaRc=897587224200000.0),
grid=namespace(Nmbpd=7,
mmin=1e-12,
mmax=100000.0,
Nr=100,
rmin=14959787070000.0,
rmax=1.495978707e+16),
star=namespace(M=1.988409870698051e+33, R=139140000000.0, T=5772.0))
Stellar parameters
The stellar parameters mainly influence the dynamical time scales and the temperature profile.
[4]:
sim.ini.star
[4]:
namespace(M=1.988409870698051e+33, R=139140000000.0, T=5772.0)
M
R
T
Grid parameters
Simulation.ini.grid
namespace to customize your mass grid. The mass grid has to be strickly logarithmic. Otherwise, the coagulation algorithm will produce wrong results.Simulation.dust.coagulation
.Note: The performance of DustPy
is very sensitive to the mass grid. A finer mass grid slows down the simulation drastically.
[5]:
sim.ini.grid
[5]:
namespace(Nmbpd=7,
mmin=1e-12,
mmax=100000.0,
Nr=100,
rmin=14959787070000.0,
rmax=1.495978707e+16)
Nmbpd
mmin
Minimum particle mass, default: \(10^{-12}\) g
mmax
Maximum particle mass, default: \(10^{5}\) g
Nr
Number of radial grid cells, default: 100
rmin
Location of inner radial grid boundary, default 1 AU
rmax
Location of outer radial grid boundary, default 1000 AU
Gas Parameters
The gas parameters define the initial conditions of the gas disk. The standard surface density profile is the self-similar solution of Lynden-Bell & Pringle (1974).
[6]:
sim.ini.gas
[6]:
namespace(alpha=0.001,
Mdisk=9.942049353490256e+31,
mu=3.847030424486999e-24,
SigmaExp=-1.0,
SigmaRc=897587224200000.0)
alpha
Mdisk
Inital gas disk mass, default: \(0.05\ M_\odot\)
mu
Mean molecular weight of the gas, default: \(2.3\ m_\mathrm{proton}\)
SigmaExp
SigmaRc
Dust Parameters
The dust parameters define the initial conditions of the dust disk and the basic collisional behavior of the dust particles.
[7]:
sim.ini.dust
[7]:
namespace(aIniMax=0.0001,
allowDriftingParticles=False,
erosionMassRatio=10.0,
d2gRatio=0.01,
distExp=-3.5,
excavatedMass=1.0,
fragmentDistribution=-1.8333333333333333,
rhoMonomer=1.67,
vFrag=100.0)
aIniMax
Maximum particle size that will be filled initially, default: 1 µm
allowDriftingParticles
True
initially drifting particles will not be removed, default: False
False
, these drifting particles will be removed.erosionMassRatio
d2gRatio
Initial dust-to-gas ratio, default: \(10^{-2}\)
distExp
Simulation.ini.dust.aIniMax
. The standard model uses the so-called MRN distribution of interstellar grains as initial condition. See Mathis et al. (1977).excavatedMass
fragmentDistribution
rhoMonomer
vFrag
Changing the Initial Conditions
In this example we want to run a simulation with a more massive disk mass. We can use the constants module of DustPy
.
[8]:
from dustpy import constants as c
[9]:
sim.ini.gas.Mdisk = 0.1 * c.M_sun
We can now initialize the simulation as described in the previous section.
[10]:
sim.initialize()
Changing the Snapshots
The standard model runs for 100,000 years and starts writing data files at 1,000 years with 10 files per time decade. In this example we only want to run the simulation for 10,000 years with only 5 snapshots per decade. This can easily be set by modifying Simulation.t.snapshots
. The starting time has to be included to write outputs for the initial conditions.
[11]:
import numpy as np
[12]:
sim.t.snapshots = np.hstack(
[sim.t, np.geomspace(1.e3, 1.e4, num=6) * c.year]
)
Changing the Output Directory
By default DustPy
will protect already existing data files. If we ran the simulation now, an error would be raised, because we have already existing data files in the output directory from the previous chapter.
We could either set Simulation.writer.overwrite
to True
to overwrite our existing data files or we could change the name of the output directory.
[13]:
sim.writer.datadir = "2_data"
The simulation is now ready to go.
[14]:
sim.run()
DustPy v1.0.7
Documentation: https://stammler.github.io/dustpy/
PyPI: https://pypi.org/project/dustpy/
GitHub: https://github.com/stammler/dustpy/
Please cite Stammler & Birnstiel (2022).
Checking for mass conservation...
- Sticking:
max. rel. error: 2.81e-14
for particle collision
m[114] = 1.93e+04 g with
m[116] = 3.73e+04 g
- Full fragmentation:
max. rel. error: 6.66e-16
for particle collision
m[90] = 7.20e+00 g with
m[95] = 3.73e+01 g
- Erosion:
max. rel. error: 1.78e-15
for particle collision
m[110] = 5.18e+03 g with
m[118] = 7.20e+04 g
Creating data directory 2_data.
Writing file 2_data/data0000.hdf5
Writing dump file 2_data/frame.dmp
Writing file 2_data/data0001.hdf5
Writing dump file 2_data/frame.dmp
Writing file 2_data/data0002.hdf5
Writing dump file 2_data/frame.dmp
Writing file 2_data/data0003.hdf5
Writing dump file 2_data/frame.dmp
Writing file 2_data/data0004.hdf5
Writing dump file 2_data/frame.dmp
Writing file 2_data/data0005.hdf5
Writing dump file 2_data/frame.dmp
Writing file 2_data/data0006.hdf5
Writing dump file 2_data/frame.dmp
Execution time: 0:01:14
The simulation has now run for 10,000 years
[15]:
sim.t / c.year
[15]:
10000.0
and we can have a look at the current state.
[16]:
from dustpy import plot
[17]:
plot.panel(sim)
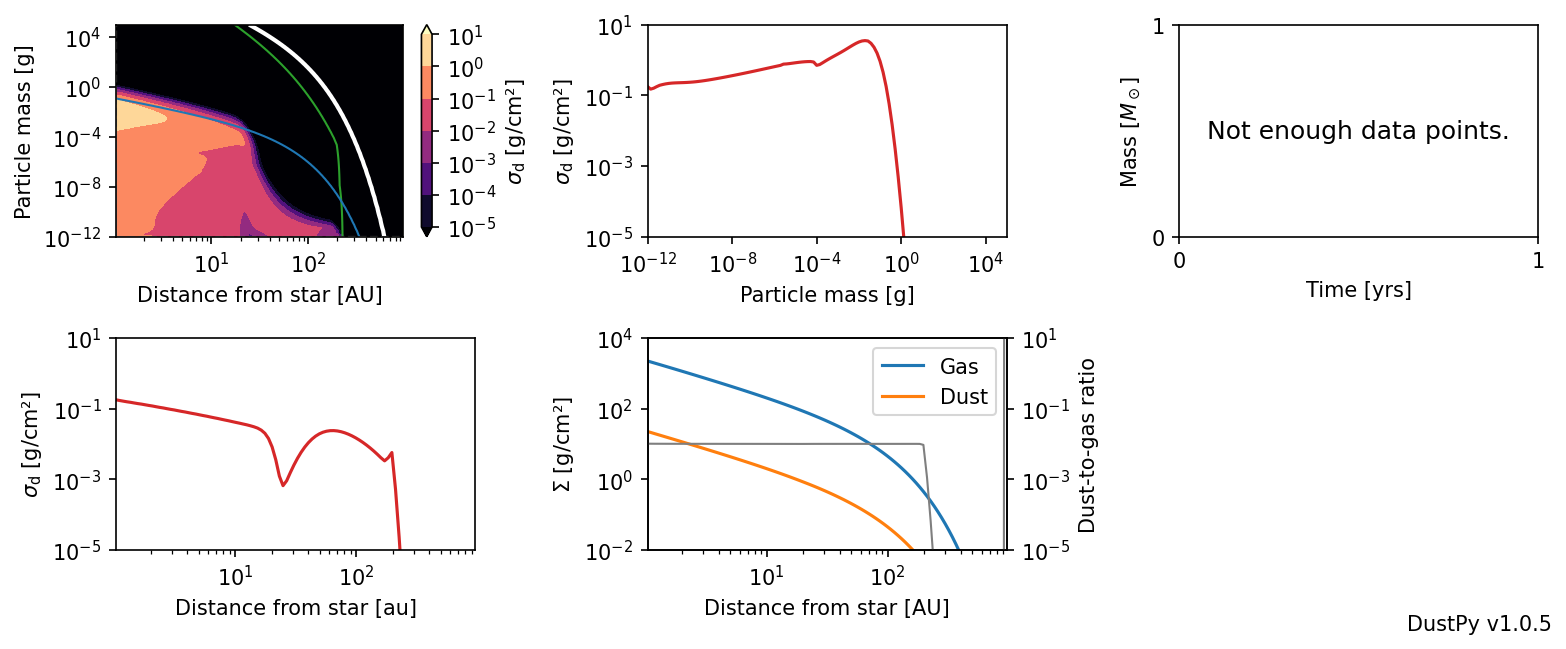
When calling Simulation.run()
, DustPy
will perform a small mass conservation check, by going through all possible collisions and calculating their relative mass error. The limitation here is machine precision. Everything smaller than \(10^{-13}\) is still acceptable. If you created your own collision model, that is not compatible with the default sticking-erosion-fragmentation model, you can either ignore the output, or you can overwrite the Simulation.checkmassconservation()
function that is called by default when calling Simulation.run()
.
See a later chapter for details on coagulation.