7. Gas Evolution
DustPy
solves gas evolution with an implicit scheme. It is solving by default the following equation for a viscously evolving disk
Where the radial gas velocity is given by
Simulation.dust.backreaction
and the viscous accretion velocity is given by
with the kinematic viscosity
[1]:
from dustpy import Simulation
sim = Simulation()
sim.ini.grid.Nr = 15
sim.initialize()
Hydrodynamics
The implicit matrix equation that is solved for gas evolution is given by
which can be written as
with
The Jacobian
[2]:
import matplotlib.pyplot as plt
import numpy as np
[3]:
fig = plt.figure(dpi=150)
ax = fig.add_subplot(111)
ax.imshow(np.where(sim.gas.Sigma.jacobian().toarray() != 0., 1., 0.), cmap="Blues")
ax.hlines(np.arange(0., sim.grid.Nr)-0.5, -0.5, sim.grid.Nr-0.5, color="gray", alpha=0.5)
ax.vlines(np.arange(0., sim.grid.Nr)-0.5, -0.5, sim.grid.Nr-0.5, color="gray", alpha=0.5)
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
ax.set_title(r"Structure of Jacobian $\mathbb{J}$")
fig.tight_layout()
plt.show()
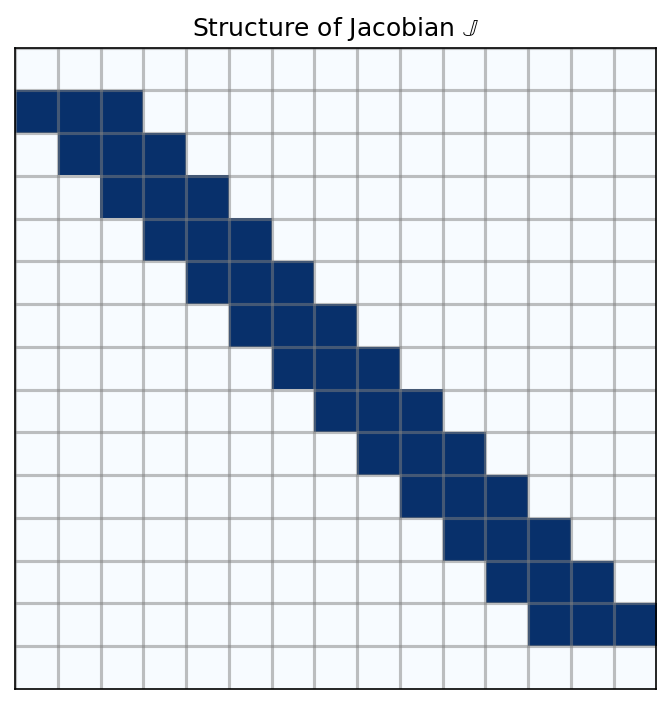
Notable exceptions are the first and the last row, which are used to set the boundary conditions. They require up to three elements to set the gradients if needed.
In the default model the inner boundary condition is set to constant gradient, which requires the following values
The outer boundary is set to floor value, which requires
The implicit integration schemes that come with simframe
only integrate equations of kind
Simulation.gas.Sigma.jacobian()
is called, the right-hand side of our equation is calculated and stored in a hidden field Simulation.gas._rhs
, that can be accessed by our integration scheme.The first and the last rows of the Jacobian, which contain the boundary conditions do not contain the time step
The custom integration scheme can be found in dustpy.std.gas.impl_1_direct
.
The hydrodynamic source terms can be calculated with a matrix multiplication of the Jacobian with the gas surface density.
[4]:
sim.gas.Sigma.jacobian() @ sim.gas.Sigma
[4]:
array([ 0.00000000e+00, -1.03359588e-11, -4.51471707e-12, -2.69067755e-12,
-1.54946950e-12, -8.43606966e-13, -4.18106923e-13, -1.75513252e-13,
-5.25046677e-14, -3.97896651e-15, 5.72327978e-15, 2.97210931e-15,
5.76779101e-16, 4.02570131e-17, 0.00000000e+00])
However, the first and the last element will not have the correct value, since they contain the instructions for the boundaries without the time step.
The interface fluxes and the gas velocity will be calculated after the integration step, when the new values of the gas surface density will be accessible. Changes to the fluxes or the gas velocities will therefore not influence the gas evolution. In other words, you cannot turn off the hydrodynamic gas evolution by setting the viscous velocity to zero.
Turning off Hydrodynamics
The diagonals of the Jacobian contain information about the grid
Note: This is only true, if there is no backreaction, i.e.,
[5]:
sim.gas.nu[:] = 0.
sim.gas.nu.updater = None
[6]:
sim.gas.Sigma.jacobian() @ sim.gas.Sigma
[6]:
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
Another option would be to set the Simulation.gas.alpha
to zero and unset its updater. This would, however, influence other fields that depend on
External Sources
The external gas sources Simulation.gas.S.ext
can be used as additional source or loss term, for gas infall or photoevaporation. By default they are set to zero.
The first and the last element of the array will not have an effect on gas evolution, since they will be overwritten by the integration scheme to set the boundaries as described above.
Turning off External Sources
To turn off external source terms set the field to zero and unset its updater.
[7]:
sim.gas.S.ext[:] = 0.
sim.gas.S.ext.updater = None
Turning off Gas Evolution
To turn off the entire gas evolution you can either turn off hte individual parts as described above or you can remove the integration instruction of the gas from the instruction list.
[8]:
sim.integrator.instructions
[8]:
[Instruction (Dust: implicit 1st-order direct solver),
Instruction (Gas: implicit 1st-order direct solver)]
[9]:
del(sim.integrator.instructions[1])
[10]:
sim.integrator.instructions
[10]:
[Instruction (Dust: implicit 1st-order direct solver)]
If you simply remove the integration instruction, the gas velocities will still be calculated at every time step, since the viscosity is non-zero. This will therefore still influence dust quantities that require the gas velocity.